Firstly, I create a concrete Quest, SlayDragonQuest, and I let Spring know, through annotations, that it is a component and it is qualified as quest.
@Component @Qualifier("quest") public class SlayDragonQuest implements Quest { public String embark() { return "Embarking on quest to slay the dragon!"; } }Then I modify my BraveKnight to let Spring know that it is a component too, named knight, and that it is autowired to the component that is qualified as quest.
@Component("knight") public class BraveKnight implements Knight { // ... @Autowired public BraveKnight(@Qualifier("quest") Quest quest) { this.quest = quest; } // ... }Let's have a look to the KnightController.
@RestController // 1 public class KnightController { @Resource private Knight knight; // 2 @RequestMapping("/quest") public String quest() { // 3 return knight.doQuest(); } }1. It is annotated as a RestController, so Spring would use it as a RESTful service.
2. Its knight field is annotated as resource, so Spring would try to inject in it a component named as its type (but starting with a lowercase letter), meaning, the BraveKnight.
3. Any request to the /quest URI is mapped to this method.
I only have to run it:
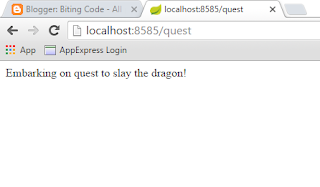
If something is not clear on this last step, you may get some hints from the previous Hello Spring Boot post.
I have written this code while reading the first chapter of Spring in Action, Fourth Edition by Craig Walls. It diverges a bit from the original version, since I have developed a Maven Spring Boot Web project, and here I have used annotations instead of the classic xml configuration to signal beans to Spring and wire them together.
Full code on github.
No comments:
Post a Comment